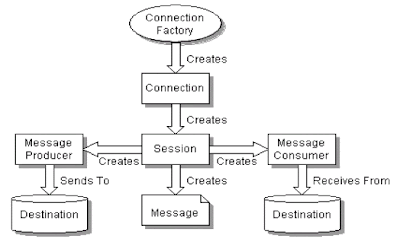
JMS : Java Messaging Service
I was searching an easy and running example on JMS, but could not. I saw in many tutorial they explained in very hard manner to how to setup the administrative object to run the JSM example. But in real it is very easy.
Here I am using IBM Rational Software Architect (RSA) as Java IDE and WebSphere Application Server V6.1 that comes with RSA. [ Image Source ]
I was searching an easy and running example on JMS, but could not. I saw in many tutorial they explained in very hard manner to how to setup the administrative object to run the JSM example. But in real it is very easy.
Here I am using IBM Rational Software Architect (RSA) as Java IDE and WebSphere Application Server V6.1 that comes with RSA. [ Image Source ]
NOTE : If you want to execute this tutorial on RAD [IBM Rational Architect Developer] IDE then plesae click below link.
Just follows these step and your example will run:
1. start the server and go to admin console
2. Service Integration -> Buses -> New -> Give Bus Name: BinodBus ->
Next -> Finish
3. click on BinodBus -> In Topology Section, click on Bus Member -> Add -> next -> Chosse File Store -> next -> next -> Finish -> Save
4. Agin click on BinodBus -> In Destination Resource, click on Destination -> check Queue Type present or not. If not present then click on Next -> Choose Queue -> Next ->
put Identifier QueueDestination -> Next -> Finish -> Save
5. Open Resources Tree from left panel
6. click on JMS -> Connection Factories -> New -> Choose Default messaging provider -> OK -> Name -> BinodConnectionProvider ->
JNDI Name -> jms/BinodConnectionProvider -> Bus Name -> BinodBus ->
click on OK -> Save
7. From left side Resources -> JMS -> Queue -> New -> choose Default messaging provider -> OK ->
Name -> BinodQueue -> JNDI -> jms/BinodQueue -> Bus Name ->
BinodBus -> QueueName -> QueueDestination -> OK -> Save
[All the bold latter is Admin object name]
8. Restart the server.
9. Create one Dynamic Web Project (JMSSECOND)and Write two servlet to check the simple example
10. Write first servlet (ProducerServlet.java)
import java.io.IOException;
import javax.jms.Connection;
import javax.jms.ConnectionFactory;
import javax.jms.Destination;
import javax.jms.JMSException;
import javax.jms.MessageProducer;
import javax.jms.Session;
import javax.jms.TextMessage;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ProducerServlet extends javax.servlet.http.HttpServlet implements javax.servlet.Servlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("******** THIS IS MESSAGE PRODUCER SERVLET **********");
check();
}
public void check(){
System.out.println("********* Producer check **********");
String destName = "jms/BinodQueue";
final int NUM_MSGS = 5;
Context jndiContext = null;
try { jndiContext = new InitialContext(); }
catch (NamingException e) { System.out.println("Could not create JNDI API context: " + e.toString()); System.exit(1);
}
ConnectionFactory connectionFactory = null;
Destination dest = null;
try {
connectionFactory = (ConnectionFactory) jndiContext.lookup("jms/BinodConnectionProvider");
dest = (Destination) jndiContext.lookup(destName); }
catch (Exception e) { System.out.println("JNDI API lookup failed: " + e.toString()); e.printStackTrace(); System.exit(1);
}
Connection connection = null;
MessageProducer producer = null;
try {
connection = connectionFactory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); producer = session.createProducer(dest);
TextMessage message = session.createTextMessage();
for (int i = 0; i < NUM_MSGS; i++) {
message.setText("This is message from JMSSECOND DEMO " + (i + 1));
System.out.println("Sending message: " + message.getText());
producer.send(message);
}
producer.send(session.createMessage());
} catch (JMSException e) { System.out.println("Exception occurred: " + e.toString()); }
finally { if (connection != null) { try { connection.close(); }
catch (JMSException e) { }
}
}
}
}
11. Write second servlet (ConsumerServlet.java)
import java.io.IOException;
import javax.jms.Connection;
import javax.jms.ConnectionFactory;
import javax.jms.Destination;
import javax.jms.JMSException;
import javax.jms.Message;
import javax.jms.MessageConsumer;
import javax.jms.Session;
import javax.jms.TextMessage;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ConsumerServlet extends javax.servlet.http.HttpServlet implements javax.servlet.Servlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("********** MESSAGE CONSUMER SERVLET 2 ************");
check();
}
public void check(){
System.out.println("********* Consumer check **********");
String destName = "jms/BinodQueue";
Context jndiContext = null;
ConnectionFactory connectionFactory = null;
Connection connection = null;
Session session = null;
Destination dest = null;
MessageConsumer consumer = null;
TextMessage message = null;
System.out.println("Destination name is " + destName);
try {
jndiContext = new InitialContext();
}catch (NamingException e) { System.out.println("Could not create JNDI API context: " + e.toString()); System.exit(1);
}
try {
connectionFactory = (ConnectionFactory) jndiContext.lookup("jms/BinodConnectionProvider");
dest = (Destination) jndiContext.lookup(destName);
} catch (Exception e) { System.out.println("JNDI API lookup failed: " + e.toString()); System.exit(1);
}
try {
connection = connectionFactory.createConnection();
session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
consumer = session.createConsumer(dest);
connection.start();
while (true) {
Message m = consumer.receive(1);
if (m != null) {
if (m instanceof TextMessage) {
message = (TextMessage) m;
System.out.println("Reading message: " + message.getText()); }
else { break; }
}
}
} catch (JMSException e) { System.out.println("Exception occurred: " + e.toString()); }
finally { if (connection != null) { try { connection.close(); }
catch (JMSException e) { }
}
}
}
}
First run Producer Servlet:
http://localhost:9080/JMSSECOND/ProducerServlet
Output:
Sending message: This is message from JMSSECOND DEMO 1
Sending message: This is message from JMSSECOND DEMO 2
Sending message: This is message from JMSSECOND DEMO 3
Sending message: This is message from JMSSECOND DEMO 4
Sending message: This is message from JMSSECOND DEMO 5
Then run Consumer Servlet:
http://localhost:9080/JMSSECOND/ConsumerServlet
Output:
Reading message: This is message from JMSSECOND DEMO 1
Reading message: This is message from JMSSECOND DEMO 2
Reading message: This is message from JMSSECOND DEMO 3
Reading message: This is message from JMSSECOND DEMO 4
Reading message: This is message from JMSSECOND DEMO 5
Please put your comments. I am able to write this article after a lot struggle.
Source of example.
Woohoo awesome job. First time I got an easy example on JMS. Any one can start JMS with this easy example. Thanks a lot.
ReplyDeleteSachin, HYD
Thanks for your appreciation and keeping hope for your suggestion to improve this blog.
ReplyDeleteThanks,
Binod Suman
http://binodsuman.blogspot.com
Good tutorial. I have also got one more good link http://sacrosanctblood.blogspot.com/2008/11/jms-tutorial-topic-subscriber-client.html
ReplyDeleteThanks,
Mike
Good tutorial. I have also got one more good link http://sacrosanctblood.blogspot.com/2008/11/jms-tutorial-topic-subscriber-client.html
ReplyDeleteThanks,
Mike
Thanks for taking the time to help, I really apprciate it.
ReplyDeletehttp://dealzfirst.com/Just+My+Size+JMS-3.htm
That is Good Exmple.just one more thing I want Jms with topic example could you please help me
ReplyDeleteThis is my mail-idkrishnamurthy@hcl.in
please help me
Thanks Binod.
ReplyDeleteNicely simplified example.
best regards,
Shaun D.
Hi Suman
ReplyDeleteThanks for such a good application and is very easy to understand for the beginners..
Rajesh(SE)
It really helped me a lot
ReplyDeleteHi Binod,
ReplyDeleteIts very nice job for begineers.
It will be helpfule for me,If you help me figure out JSF and Spring.
Thanks & Regards,
Jain
The most concise explanation to get started with JMS. Well done Binod Keep doing good job
ReplyDeleteSuperb tutorial.
ReplyDeleteThanks a lot
ReplyDeleteI am a bigginer to JMS. This tutorial helped me a lot . Can you pls send some more asynchronous examples
ReplyDeleteI really hope these System.exit(1) calls are not your favourite way of dealing with exceptions.
ReplyDeleteVery Nice example. It came the first result on google for my search. Thanks very much:)
ReplyDeleteReally good one..thanks a lot, I went through so many articles to understand this simple thing!!
ReplyDeleteHi,
ReplyDeleteMany thanks for your great tutorial.
One question; how can we connect to a remote websphere?
What are the code changes for this purpose?
Thanks, Mr. Binod Suman, I have tested it successfully on glassfish 3.0.1. Keep up the good work!
ReplyDeleteThaks.
ReplyDeleteBut i have some problem when is use security for bus.
How for do it.
thank for reply.
Hai its nice example! could u tell us how to deploy jms on weblogic8.1......
ReplyDeleteHi Binod,
ReplyDeleteReally nice work this.
I migrated your example to Weblogic 10g in a Java Main class. Really appreciate your work. It made me understand the basic working of JMS.
Siddharth Singh
th
ReplyDeleteHi binod
ReplyDeletewhen i am trying this get error like
[7/21/11 15:13:38:890 IST] 00000052 SibMessage E [:] CWSIQ0017E: The sender channel MQ_SENDER_CHANNEL for MQLink TESTSIB_LINK has failed to establish a connection with the remote host localhost/127.0.0.1 because the remote listener for port 2020 is not available.
how can i rectify
Its once of the fantastic blog i ever seen. Its very simple and very use full. Its really good for evry level of developer.
ReplyDeleteWonderful, too good.I simply loved the example
ReplyDeleteThis is a good and a pragmatic article on JMS. It is very helpful in understanding the workings of JMS.
ReplyDeleteThank you
Hi Binod,
ReplyDeleteReally, this is good example.
I appreciate you.
Thanks
SinghRaj
Princesscruises
Thank you for this example!
ReplyDeleteThanks! After long time at last have found a working example without EJB. Good one really.
ReplyDeleteThanks a lot!
Good work Binod.
ReplyDeleteIts really good example..
BestRegards,
Suresh Mopada
Thanks Binod, This has been one of the best article for the JMS Beginner.
ReplyDeleteTribhuwan
explanation is good ... thank you
ReplyDeleterielly rielly good tutorial
ReplyDeleteNice work . Thanks a lot
ReplyDeleteReally Good tutorial. I was able to setup the example exactly as described. Thanks a lot.
ReplyDeleteI made a couple of tweaks to the example in my version so it was a little easier to see.
At the moment the output goes to the log file and not the web page, so I added a println() method which writes to both. That way the user gets something on their web browser and also in the log file.
Changed code from :
check();
}
public void check() {
Changed to:
check(response.getWriter());
}
public void println(PrintWriter out,String message){
System.out.println(message);
out.println("<p>");
out.println(message);
out.println("</p>");
}
public void check(PrintWriter out) {
Then change each :
System.out.println("some text");
changed to :
println(out,"some text at "+System.currentTimeMillis());
Very simple and easy to understand
ReplyDeleteVery Nice ....
ReplyDeleteExactly what I was looking .... Thank you very much for putting this together.
thank vry much Binod.please send me documentation on jms to khaja.sendme@gmail.com
ReplyDeleteHi Binod,
ReplyDeleteThanks for the above examples
It would be great if you can help me out with a java program which monitors a queue continuously. Thanks in advance.
Ajith
Nice tutorial. Easy to start JMS
ReplyDeletegood example .thamk you.
ReplyDeletethanks for the link to your tool.Amc Square Reviews
ReplyDeleteThanks for the link to your tool.Amc Square Reviews
ReplyDelete